How to capture HTTPS traffic from Flutter iOS app
1. Flutter and network calls
Flutter is a popular framework from Google that helps developers build apps for iOS, Android and other platforms using a single codebase.
When building apps with Flutter, developers often need to make network calls to fetch data from servers or send data to them. However, these network calls can sometimes fail or not work as expected. Being able to see and inspect the actual HTTP/HTTPS traffic between your app and servers is very helpful for finding and fixing issues during development.
2. How to capture HTTPS traffic from Flutter iOS app with Proxyman
2.1 Add HTTP Proxy Configuration to Flutter
By default, Flutter does not use any HTTP proxy configuration. You need to manually add the HTTP proxy configuration to your Flutter project.
It depends on what HTTP Network library you are using in your Flutter project. For this example, we will use http
package.
Make sure to replace <YOUR_LOCAL_IP>
with the external IP of your computer if you're using Android. You can get the IP in Proxyman -> Tools Menu -> Install for iOS -> Physical Device
http
package
// Make sure to replace <YOUR_LOCAL_IP> with // the external IP of your computer if you're using Android. // You can get the IP in the Android Setup Guide window String proxy = Platform.isAndroid ? '<YOUR_LOCAL_IP>:9090' : 'localhost:9090'; // Create a new HttpClient instance. HttpClient httpClient = new HttpClient(); // Hook into the findProxy callback to set // the client's proxy. httpClient.findProxy = (uri) { return "PROXY $proxy;"; }; // This is a workaround to allow Proxyman to receive // SSL payloads when your app is running on Android. httpClient.badCertificateCallback = ((X509Certificate cert, String host, int port) => true); // Pass your newly instantiated HttpClient to http.IOClient. IOClient myClient = IOClient(httpClient); // Make your request as normal. var response = myClient.get('/my-url');
Dio library
// Make sure to replace <YOUR_LOCAL_IP> with // the external IP of your computer if you're using Android. // You can get the IP in the Android Setup Guide window String proxy = Platform.isAndroid ? '<YOUR_LOCAL_IP>:9090' : 'localhost:9090'; // Create a new Dio instance. Dio dio = Dio(); // Tap into the onHttpClientCreate callback // to configure the proxy just as we did earlier. (dio.httpClientAdapter as DefaultHttpClientAdapter).onHttpClientCreate = (client) { // Hook into the findProxy callback to set the client's proxy. client.findProxy = (url) { return 'PROXY $proxy'?; }; // This is a workaround to allow Proxyman to receive // SSL payloads when your app is running on Android. client.badCertificateCallback = (X509Certificate cert, String host, int port) => true; }
Dart HTTP package
// Make sure to replace <YOUR_LOCAL_IP> with // the external IP of your computer if you're using Android. // You can get the IP in the Android Setup Guide window String proxy = Platform.isAndroid ? '<YOUR_LOCAL_IP>:9090' : 'localhost:9090'; // Create a new HttpClient instance. HttpClient httpClient = new HttpClient(); // Hook into the findProxy callback to set // the client's proxy. httpClient.findProxy = (uri) { return "PROXY $proxy;"; }; // This is a workaround to allow Proxyman to receive // SSL payloads when your app is running on Android httpClient.badCertificateCallback = ((X509Certificate cert, String host, int port) => true);
You can find more the technical details in the Proxyman Flutter Documentation
2.2 Capture HTTPS/HTTP traffic from Flutter - iOS Simulator
- Open your iOS Simulator first. Make sure it's the simulator you're using to run your Flutter app.
- Let's open Proxyman -> Certificate menu -> Install for iOS -> Simulator -> Follow the steps -> It auto set the Proxy and install the certificate in 1 click
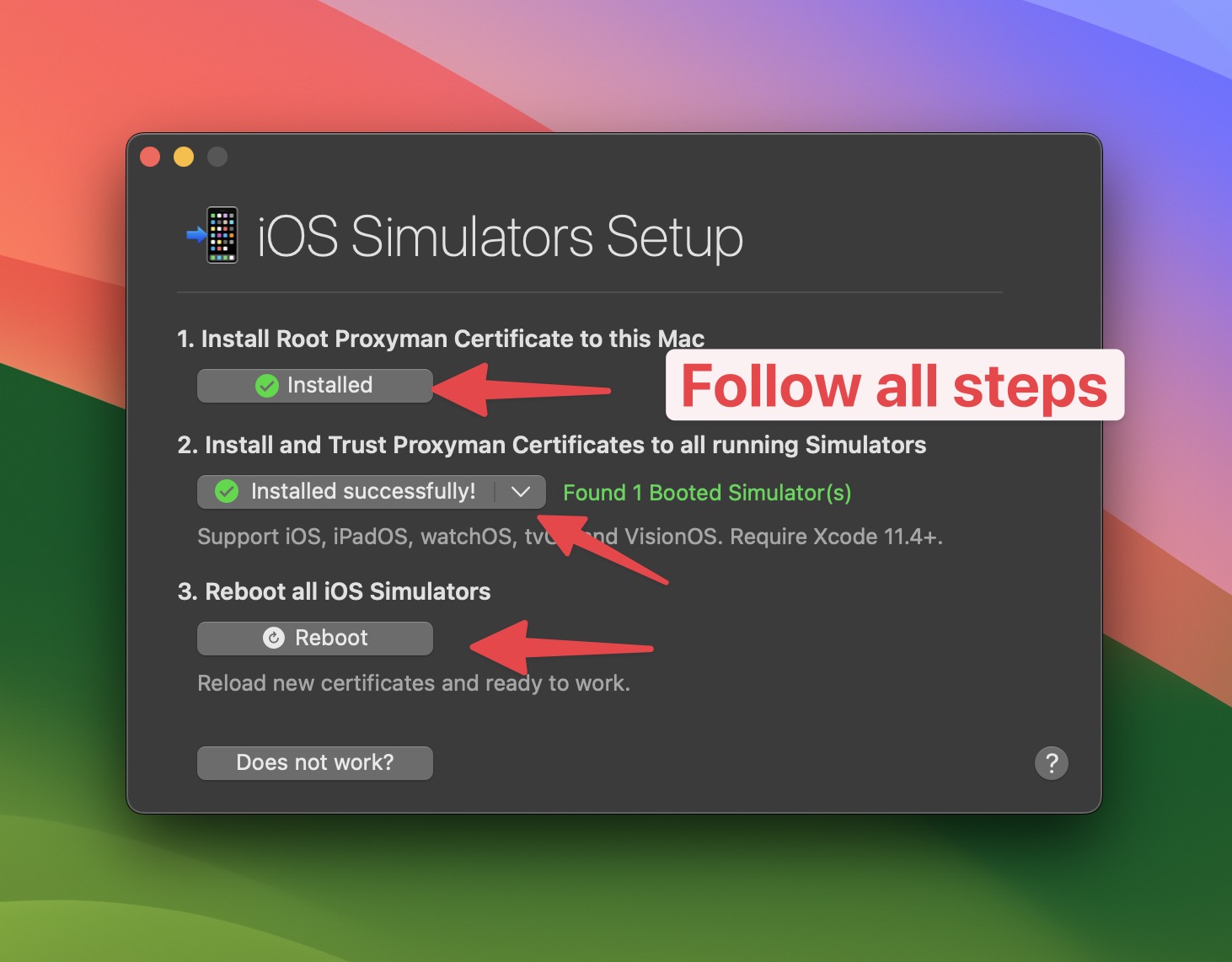
At this point, it's time to run your Flutter app on iOS Simulator and start capturing HTTP/HTTPS traffic.
Proxyman will automatically start capturing the HTTP/HTTPS traffic from your Flutter app.
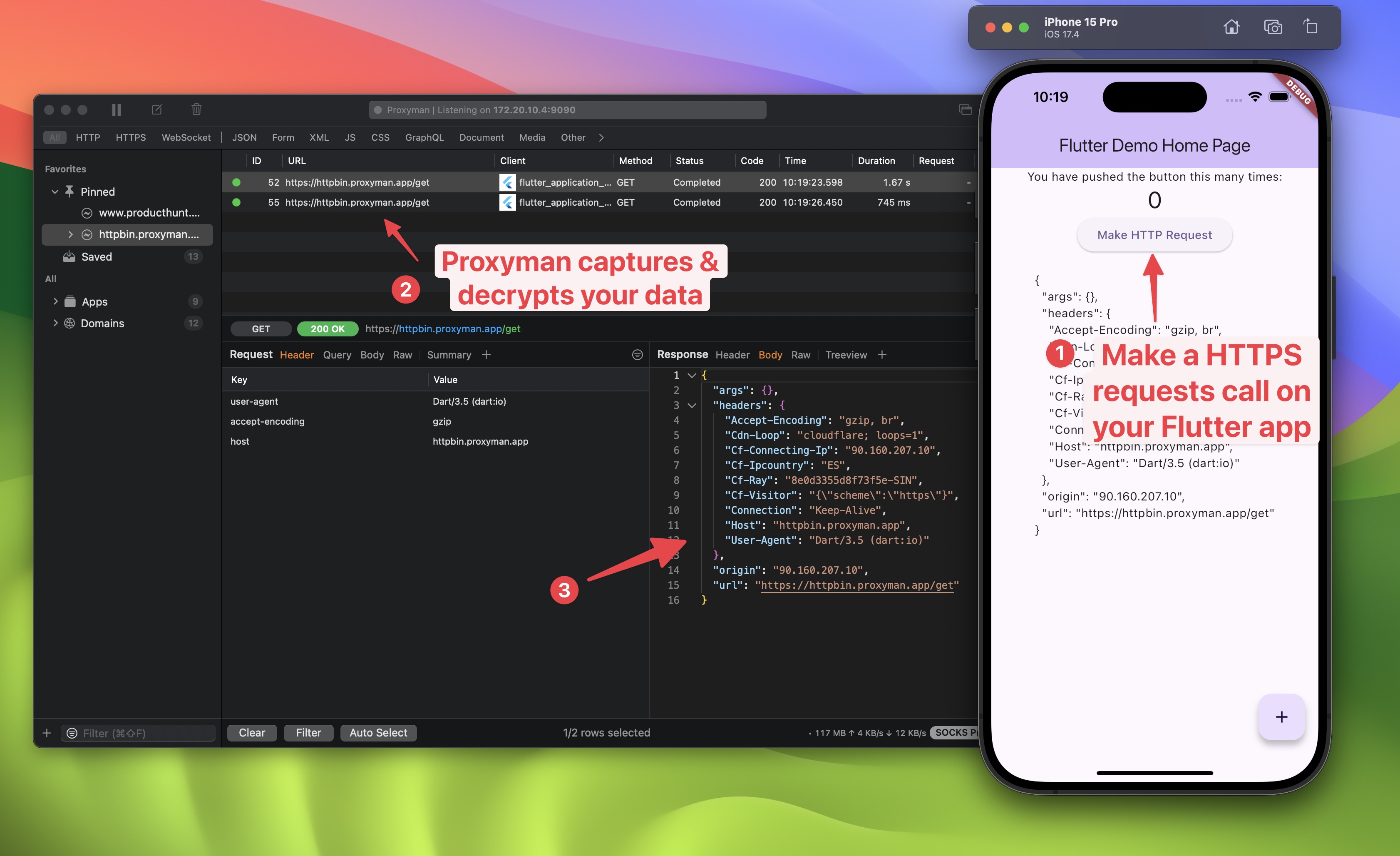
2.3. Capture HTTPS/HTTP traffic from Flutter - iOS Devices
To capture HTTP/HTTPS traffic from your Flutter app on an iOS/iPadOS device, you need to install the Proxyman certificate on the device.
Let's follow the steps in Proxyman -> Certificate menu -> Install for iOS -> Physical Device -> Follow the steps
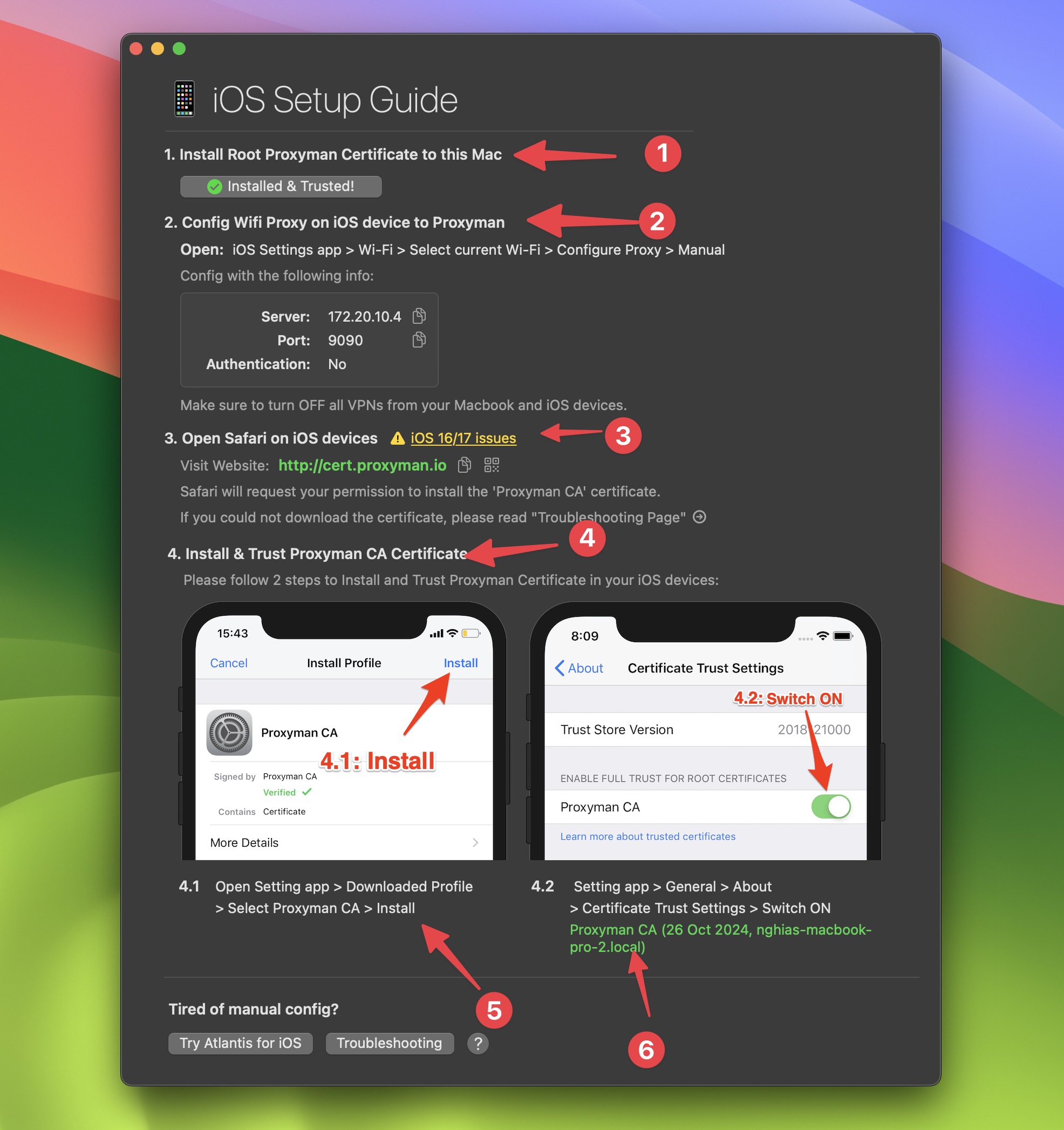
Make sure to install & trust the certificate in the last step.
At this point, you can run your Flutter app on iOS/iPadOS device and start capturing HTTP/HTTPS traffic.
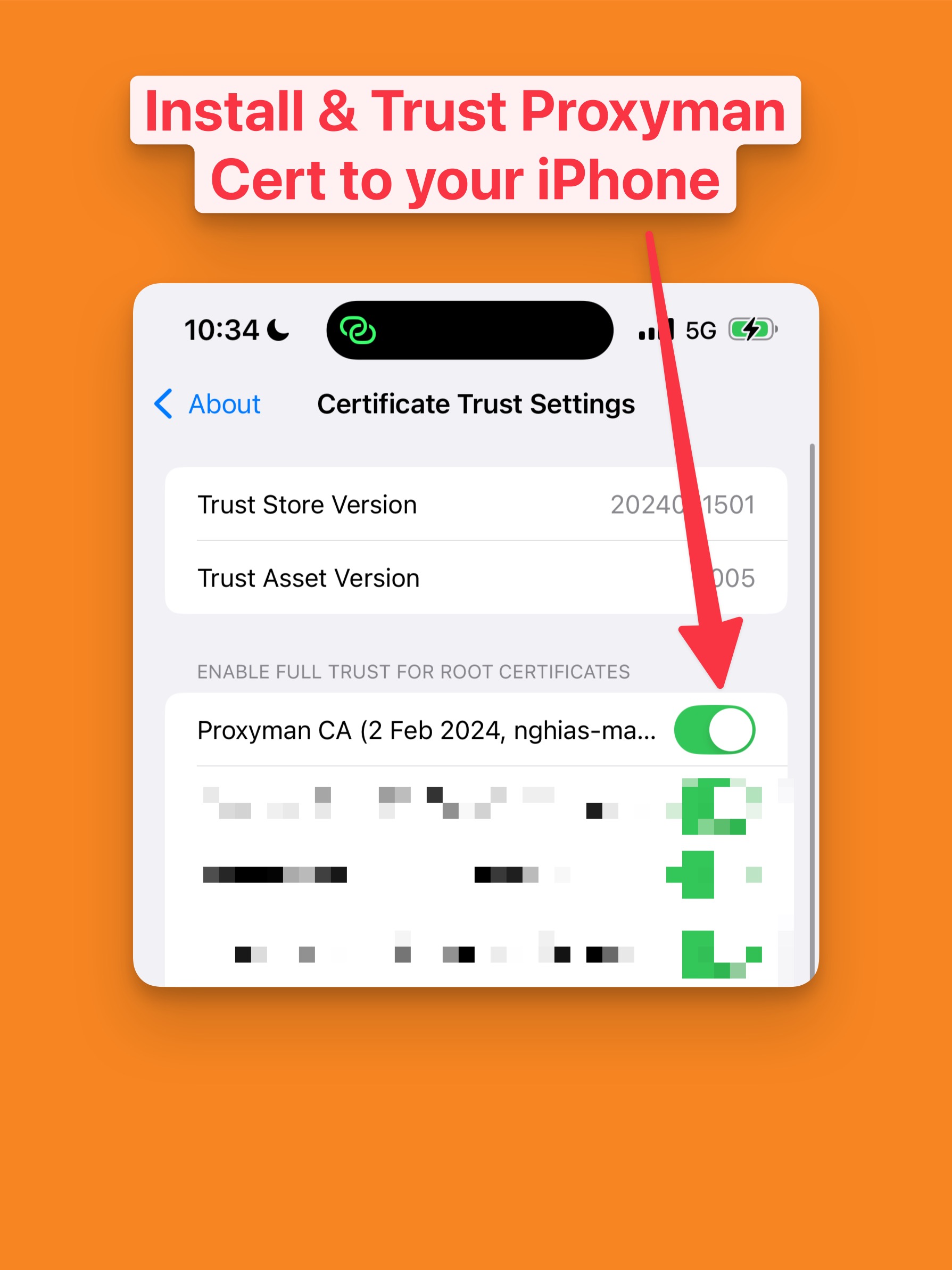
Proxyman will automatically start capturing the HTTP/HTTPS traffic from your Flutter app.
Conclusion
To capture HTTP/HTTPS traffic from your Flutter app, it's very simple.
- Add the HTTP proxy configuration to your Flutter project
- Install the Proxyman certificate on your iOS/iPadOS device
- Run your Flutter app and start capturing HTTP/HTTPS traffic with Proxyman
What's next?
- Try to use some debugging tools to inspect and modify the request & response payloads.
- Download Proxyman at Proxyman Website
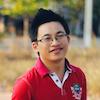